This page was generated from `/home/lectures/exp3/source/notebooks/L11/Diffraction.ipynb`_.
Diffraction¶
[2]:
import numpy as np
import matplotlib.pyplot as plt
from time import sleep,time
from ipycanvas import MultiCanvas, hold_canvas,Canvas
import matplotlib as mpl
import matplotlib.cm as cm
%matplotlib inline
%config InlineBackend.figure_format = 'retina'
# default values for plotting
plt.rcParams.update({'font.size': 12,
'axes.titlesize': 18,
'axes.labelsize': 16,
'axes.labelpad': 14,
'lines.linewidth': 1,
'lines.markersize': 10,
'xtick.labelsize' : 16,
'ytick.labelsize' : 16,
'xtick.top' : True,
'xtick.direction' : 'in',
'ytick.right' : True,
'ytick.direction' : 'in',})
Huygens Principle¶
The Huygens principle states, that each point of a wavefront is the source of a spherical wave in forward direction. This means nothing else, that any wave can be expanded into a superposition of spherical waves, which is the fundamental of Mie scattering for example. Yet, the overall statement of this principle seems a bit unphysical. Classically, accelerated charges are the source of electromagnetic waves. If there is no accelerated charge, there is no wave. Yet, the Huygens principle is in accord with quantum field theory.
Fig.: Huygens principle for a plane wave incident with a wave vector
The sketch above illustrates the Huygens principle, but we may also check that numerically. The graphs below were calculated to illustrate Huygens principle using spherical waves placed in a line close to each other.
Fig.: Huygens principle used to create a plane wave from a set of spherical waves. The graph on the left shows the amplitude of a single spherical wave of a wavelength
We have already dealt with all of the mathematics describing this situation. We have earlier looked at the interference of multiple waves with the same amplitude. In this case we have
This is again our starting point for the next calculations concernign a single slit, which confines the range of Huygens waves emitted to a region along the x-direction. This interference of Huygens waves is termed diffraction, yet the physical principle behind is interference. Our second calculation will have a look at many slits arranged along the x-direction, which is then called a diffraction grating. The latter is an indispensible tool for spectroscopy.
Single Slit diffraction¶
We would like to apply this formula to study the diffraction of an incident plane wave (wavevector
Fig.:
We will devide the slit into segments of width
where we can replace the
Substituting
then gives
Since we do not have a finite number
and therefore
where
Single Slit Diffraction
The intensity distribution generated by the diffraction of monochromatic light on a single slit and observed in the far field is given by
where
Fig.: Total wave amplitude behind a slit (b=2µm) for an incident wave of 532 nm wavelength. The plot in the middle shows the intensity in the space behind the slit. The graph on the right displays the diffraction pattern at a screen at 100 µm distance from the slit.
Let’s have a look at some of the properties of the intensity distribution with the help of some python code.
Function definition
[3]:
def single_slit(d,theta,wl):
d=np.pi*d/wl*np.sin(theta)
return((np.sin(d)/d)**2)
Parameter definition
[4]:
wl=532e-9
b=5e-6
theta=np.linspace(-np.pi/6,np.pi/6,1000)
Plotting
[11]:
plt.figure(figsize=(15,5))
plt.subplot(1,2,1)
plt.plot(np.sin(theta),single_slit(b,theta,532e-9),'green',lw=2,label="532 nm")
plt.plot(np.sin(theta),single_slit(b,theta,700e-9),'red',lw=2,label="700 nm")
plt.annotate('first minimum 700 nm',xy=(0.14, 0),xytext=(0.14, 0.25),arrowprops=dict(facecolor='black', width=0.5,headwidth=6))
plt.xlabel(r'$\sin(\theta)$')
plt.ylabel('$I/I_s$')
plt.legend()
plt.subplot(1,2,2)
plt.plot(np.sin(theta),single_slit(b/2,theta,532e-9),'green',lw=2,label="532 nm")
plt.plot(np.sin(theta),single_slit(b/2,theta,700e-9),'red',lw=2,label="700 nm")
plt.xlabel(r'$\sin(\theta)$')
plt.ylabel('$I/I_s$')
plt.legend()
plt.show()
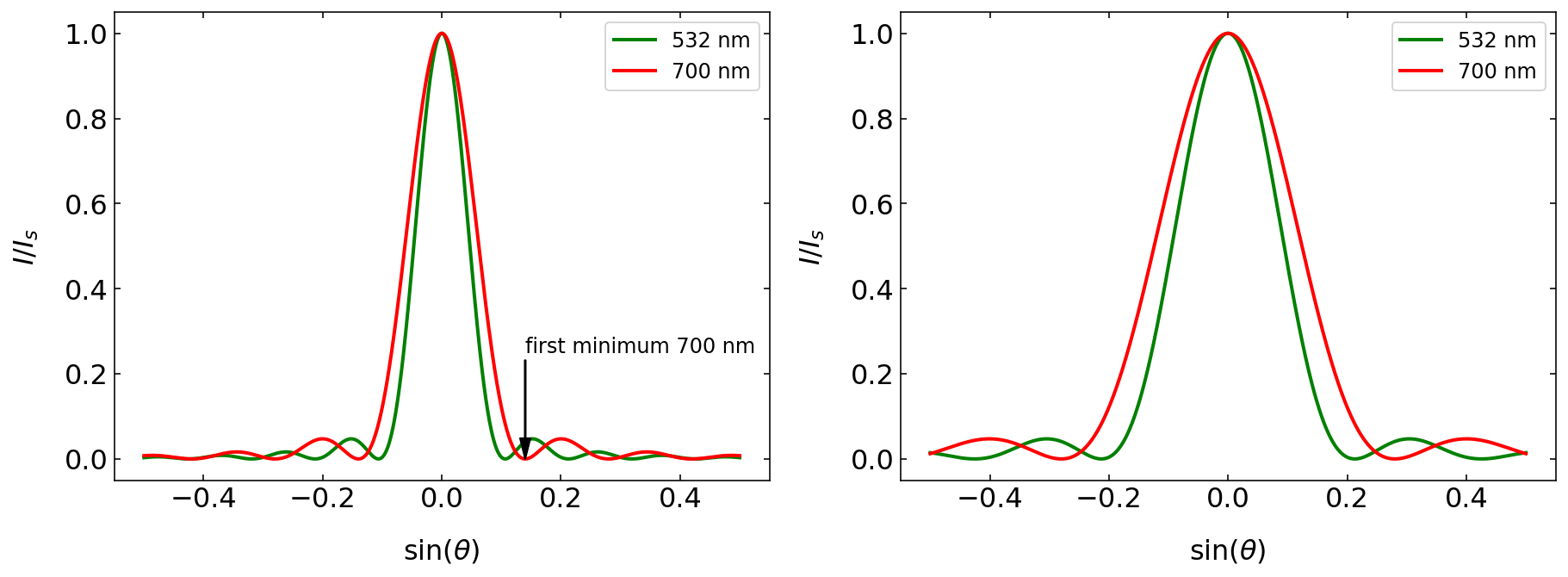
The two plots show the characteristic diffraction pattern of a single slit, which is an oscillating function, decaying in amplitude. The oscillation is defined by the numerator and the decaying amplitude by the denominator. The left graph displays the diffraction pattern as a function of
longer wavelength show a wider diffraction pattern
small slit width creates a wider diffraction pattern
We can also quantify these observation by calculating the minima of the oscillating denominator. The denomintor becomes zero whenever the argument of the sine square function is
to have a minimum in the intensity of slit diffraction. This type of dependency, i.e. wavelength divided by the dimension of the diffracting object, is valid in general even though it migh show up with diffreent numerical prefactors for other diffracting geometries.
Fig.: Diffraction patterns as a function of the sine of the diffraction angle. The minima of the diffraction pattern in this plot are at integer multiples of
Fig.: Diffraction patterns on a single slit as observed in the lecture. The left image shows the diffraction patter for red light, while the right image combines two different wavelength (red, blue), where one clearly recognizes the wider diffraction peaks for the longer red wavelength.
Circular Aperture¶
A similar but a bit more involved calculation can be done for a circular aperture. The result of this calculation is
with
where
Fig.: Diffraction pattern of a circular aperture of radius
According to the zeros of the numerator, we can again obtain the sine of the angle under which the first minimum in the intensity occurs in radial direction from the center of the diffraction pattern. We just have to set
which yields
We recognize the same dependence as before: wavelength divided by the dimension of the diffracting element. The width of the diffraction peak in the center is well defined by this first minimum. This minimum therefore defines the radius of the so called Airy disc. In the language of microscopy, it defines the size of a resolution element which is termed resel.
Diffraction on the Eye-Iris
The above description gives us some nice insight into diffraction effects which occur on the aperture of the eye - the iris. So lets do some quick estimate.
The aperture of the iris has a radius of
If we multiply this value with the diameter of the eye (roughly 2 cm), we obtain the size of the Airy disc on the “screen”, which is the retina.
So the width of the diffraction pattern of light on the retina is roughly 2.59 µm, which corresponds well to the distance between light sensitive cell in the eye around the fovea. In this region we find densities of aroiund
Resolution of an Optical Microscope¶
Resolution Definition by Reighleigh
We may spin this idea a bit further and use the diffraction pattern of a circular aperature to define the resolution of a microscope or of an optical system in general. We would like to answer the question: How close can be place two objects to be still resolved as two objects when imaged by a lens. To answer that question we first have to understand the action of a lens in wave optics. It does two things. It first of course alters the curvature of the wavefronts such that a plane wave is focused towards the focal point on the other side of the lens. The second effect ist, that the lense has a finite size, such that it can not collect all of the plane wavefront to be focused. It just cuts out a circular part. This effect corresponds to the diffraction on a circular aperture.
Fig.:
Corresponding to the above picture, we therefore create a diffraction patter for each point source on the left side. The closer the two sources come,the more will the twio diffraction patterns merge on the other side. The resolving power of a microscope may now be defined in various ways. We will refer to the definition by Rayleigh, called the Reighleigh criterion, sketched below.
Fig.: $.
The Rayleigh criterion defines two objects as still resolvable, if the maximum of the second diffraction pattern is located at the first minimum of the first diffraction pattern (see sketch above). In this case the sum of the two intensity distribution will have a minimum between the two peaks, where the intensity is reduced by 26%. Note that in this case we refer to two light sources which are incoherent, such that we do not have to consider interference.
According to the figure above, the diffraction angle for the first minimum is given by
In the case of small angles, we can approximate
where d is the distance between
On the other side, we know from the imaging equation, that
Combining both equations will yield
where
and
Rayleigh resolution criterium
The Rayleigh resolution criterium states, that two incoherent light sources can still be resolved as two independent sources, if their distance
The numerical aperture is given by
Loosly speaking you may see this formula as the following. If light emerges from point
Resolution Definition by Abbe
While we considered before the spacing between two diffraction patterns resulting from wo incoherent point-like light sources. The definition of the resolution by Abbe refers to two coherent light sources. If we have two coherent light sources, we cannot just sum up both intensity distributions, but we have to calculate the total amplitude first before we may determine the total intensity.